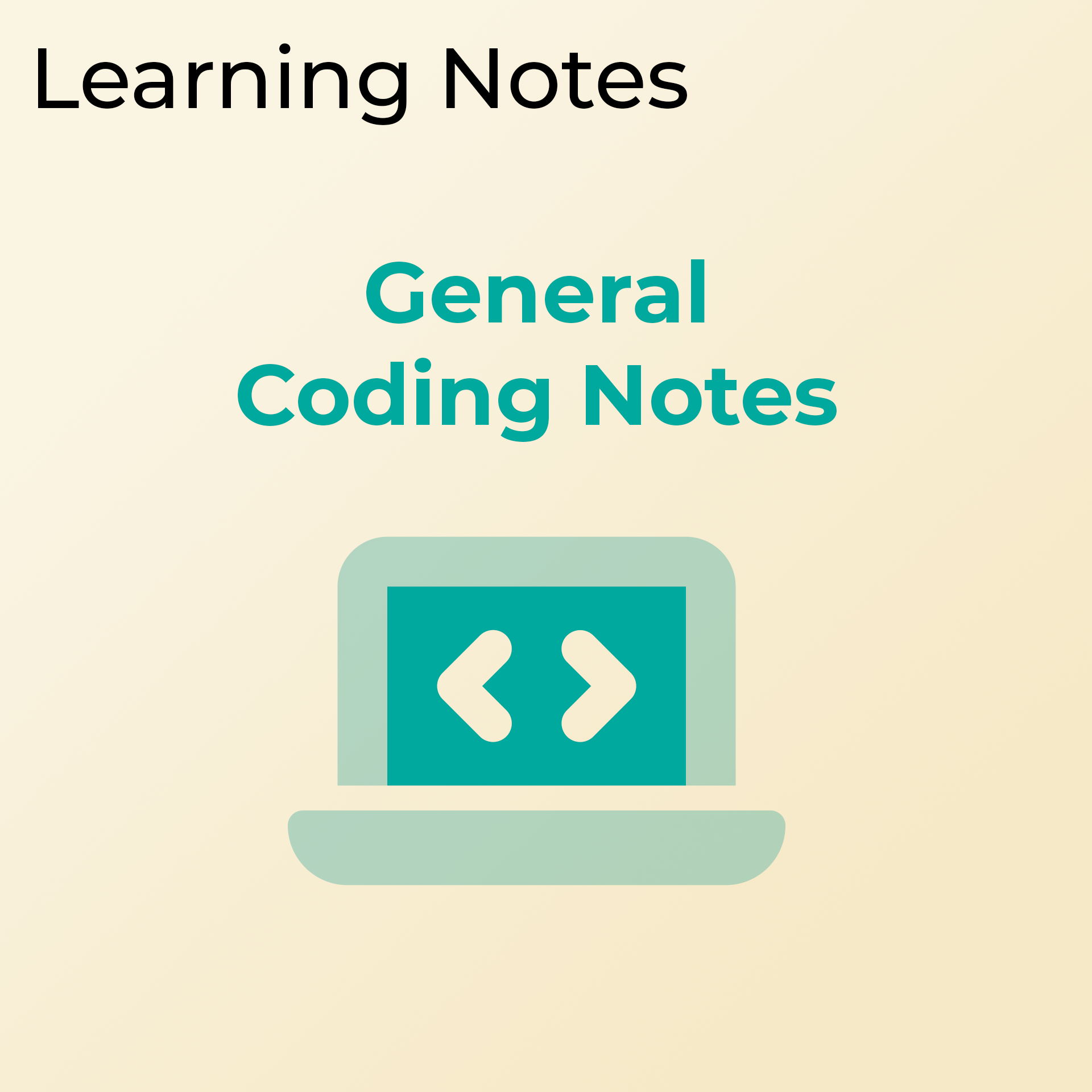
1. Coding Conventions
Give variables and functions meaningful names.
Wrong | Correct |
---|---|
int a = 100; | int health = 100; |
function b() {} | function main() {} |
Variable name conventions:
This eases the identification of variables’ data types and scope regardless of the IDE a person uses.
Constants in FULL CAPS
const string NAME = 'John Doe';
Denote private variables with a leading underscore
private string _name = 'John Doe';
Indicate the variable’s data type if it’s primitive
const string S_NAME = 'John Doe';
private int _i_health = 100;
private float _f_gravity = 8.5f;
private bool _b_isDead = false;
private string _s_playerClass = 'Warrior';
Leave a comment behind the class’s ending curly brace.
This reduces confusion about ending braces, especially for files with long lines of code.
public class Player
{
} //end Player class
2. Code Styles
2.1. Default File Headers
Code
/**********************************************************************************
- File Info -
File name : file.cs
Author(s) : DAVINA Leong Shi Yun
Date Created : 3rd Oct 2015
- Contact Info -
Email : leong.shi.yun@gmail.com
Mobile : (+65) 9369 3752 [Singapore]
All content © DAVINA Leong Shi Yun. All Rights Reserved.
* Description:
*
***********************************************************************************/
HTML
<!----------------------------------------------------------------------------------
- File Info -
File name : index.html
Author(s) : DAVINA Leong Shi Yun
Date Created : 3rd Oct 2015
- Contact Info -
Email : leong.shi.yun@gmail.com
Mobile : (+65) 9369 3752 [Singapore]
All content © DAVINA Leong Shi Yun. All Rights Reserved.
* Description:
*
----------------------------------------------------------------------------------->
2.2. Code Dividers
Code
// Private Variables ------------------------------------------------------------
HTML
<!-- Private Variables ------------------------------------------------------ -->
Region
#region <Region Name>
#endregion
3. Copyrights
© Davina Leong
4. Platform Dependent Compilation
Link: http://docs.unity3d.com/Manual/PlatformDependentCompilation.html
Commonly Used:
UNITY_EDITOR
>UNITY_STANDALONE
>UNITY_WEBPLAYER
>UNITY_4_6
>UNITY_5_0
How to use:
#if UNITY_STANDALONE
#endif
6. Not Implemented Exception
throw new System.NotImplementedException();
7. Ternary Operator (?:
)
Syntax:
<variable> = (<condition>) ? <if condition is TRUE> : <if condition is FALSE>;
Example:
Screen.lockCursor = (Screen.lockCursor == false) ? true : false;